Creating a HTML Game in React Native - Part Two

Introduction
Now that we are able to show a basic 'Hello World' Canvas experiment in a React App via NX, we can now look to use the power of NX to React Native up and running.
Why use NX for React Native?
Why indeed. We need to address this now before we continue.
The way I see it, we are not using NX here to be a Mono-Repo in the sense we throw all the code of all of our applications into a big steaming vat of code; an amalgamate hulk of unreadable code where many people work on it at the same time and that has multiple points of failure and brittle co-dependencies. I still rankle at the fact a head of engineering at one of my previous places of work could not get their head out of their own pre-conceived beliefs of mono-repos and their use. I never advocate a one repo for everything approach, but that never seemed to hit home. Smh.
We use NX as a vessel with which to separate our code and share code between our Web and IOS/Android instances. After all, what's the point of React, aside from leveraging web developer skill-sets to get a head start in IOS/Android development. Well for me, a more important part of using React Native is to share code between instances of web and native code bases with minimal duplicity. I see NX as a way of allowing an Engineer to separate concerns and encourage code reusability.
OK, so back to the code.
Installing React Native Pre-Requisites
We need to have our environment set up correctly before we can continue. Assuming you are on a Mac (m1), do the following:
- Install Xcode from the App Store
Then, run the following commands via a command prompt:
- Install brew:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
- Install node, preferably via NVM:
brew install nvm
(You can get a more thorough guide here https://tecadmin.net/install-nvm-macos-with-homebrew/) - Install xCode command line tools by running:
xcode-select --install
. It is really important that you also:- Accept the T&Cs on xocde once you start
- Go to Xcode > settings > Locations and choose the xcode command line tools again and quit.
- Failing to do the above will result in an error message when doing a pod install
- Install Watchman:
brew install watchman
- Install
cocoapods
again, but usingbrew
this time, as it will install another version compatible with the architecture of your M1 CPU:brew install cocoapods
.
Setting Up React Native
Remember in Part One where we set up a React based application to host our game module? Well now we are going to do the same for React Native; a simple shell to hold our code and display it back to the user. Fancy stuff like a UI can follow.
Firstly, let's create a simple React Native Application by running the following to install the @nrwl/react-native
package to add React Native capabilities to it.
npm install @nrwl/react-native --save-dev
(Note that using npm here might give you a peer dependency issue for [email protected] for the latest library of @nrwl/[email protected]. Your choices are to use Yarn which has no such issues, or downgrade the React Package to version 18.10.0 instead until the dependencies are sorted. I did the latter for now)
To create additional React Native apps run:
✔ What name would you like to use for the application? - react-native-app
After this, the app will be build and pod install will run. Make sure you have completed all the steps in the section 'Installing React Native Pre-Requisites'.
Running the basic IOS App
Now we want to run what NX React Native has created for us. To do this:
- In the Terminal, run:
npx nx run-ios react-native-app
- The default app will then pop up.
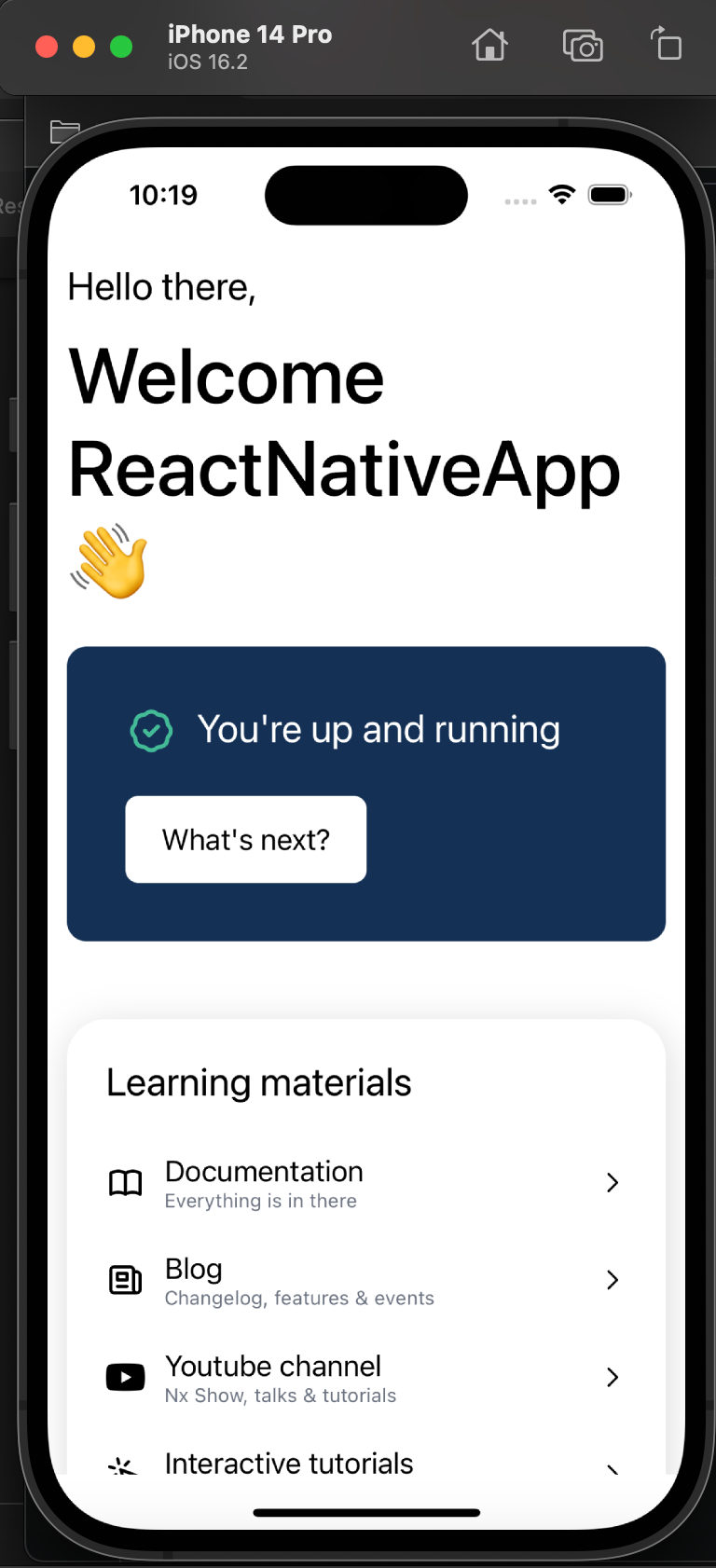
A Xcode project and workspace will also be created. You can then run this via:
- Open Xcode
- Open the workspace at ./apps/react-native-apps/ios/ReactNativeApp.xcworkspace
- Build the project by pressing the arrow in the top left corner of the screen
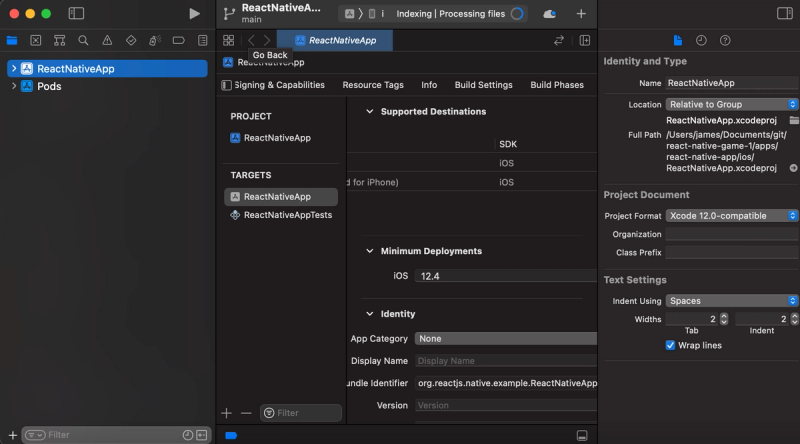
In the next part, we will discover how to modify the example and insert our own HTML example game. Remember, you can see the repo for this right here: https://github.com/jayjaybeeuk/react-native-game-1